New <form> Functionality
- New
<form>
attributesHTML5 defines two new
<form>
attributes, "autocomplete" which allows drop-down suggestion lists like Google and "novalidate" which, as its name implies, turns off validation for a form. - New
<fieldset>
attributes- "disable" - disables the
<fieldset>
- "name" - specifies the name of the
<fieldset>
- "form" - since
<fieldset>
s do not have to be nested inside their parent<form>
, this attribute links the<fieldset>
to its parent<form>
.
- "disable" - disables the
- New
<label>
attributeA label can now have a
<form>
element showing the label's parent form, since the label no longer has to reside inside it's parent<form>
- New
<input>
attribute.Like
<fieldset>
and<label>
, an<input>
element can be outside it's parent in HTML5, so it has a<form>
attribute to connect it to its parent<form>
.
New <input>
types
Instead of attaching JavaScript to verify user-input, HTML5 has baked-in commonly used functions into new input types. The new input types are:
color
,
date
,
datetime-local
,
datetime
,
email
,
month
,
number
,
range
,
search
,
tel
,
time
,
url
,
and
week
.
You can start using these now, since browsers treat a <input type="email"> or other unknown type as simply "text".
Let's create a sample form using the text input type with new attributes.
We will start with the name in a fieldset:
This is produced by the following HTML5:
<form id="signUpForm" autocomplete="on" action="javascript:myAction();" method="get"> <fieldset> <legend>About You</legend> <p> <label for="name">Name: <input id="name" name="name" type="text" placeholder="Jane Doe" autofocus required size="30" /> </label> </p> </fieldset> </form>
The placeholder
text fills the entry box with a hint, that goes away when the user starts to enter data. If the browser doesn't recognize placeholder
, it will disregard it.
autofocus
puts the focus into this box so the user doesn't have to click into the box to start typing.
required
will force the user to enter something before we can move on.
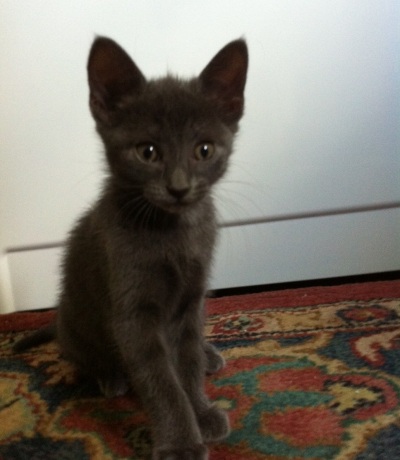
Now, let's write a form for your new "Kitten-A-Month" Club, where you can send cat lovers a kitten a month.
This is produced by the following HTML5:
<!-- from css file: input:valid { background-color: #aaffaa; } input:invalid { background-color: #ffaaaa; } --> <form id="signUpForm1" autocomplete="on" action="javascript:myAction();" method="get"> <fieldset> <legend> About You </legend> <p> <label for="name">Name:</label> <input type="text" required placeholder="Jane Doe" pattern="(.{2,30})" id="myName" name="myName" size="20" /> </p> <p> <label for="telephone">Your Telephone (XXX-XXX-XXXX):</label> <input type="tel" pattern="([0-9]{3})(-[0-9]{3})(-[0-9]{4})" id="telephone" name="telephone" placeholder="512-867-5309" required size="12" /> </p> <p> <label for="email"> Email:</label> <input type="email" placeholder="janedoe@gmail.com" id="email" name="email" required size="20" /> </p> <p> <label for="date">When do you want us to start sending you kittens?:</label> <input type="date" name="date" id="date" /> </p> </fieldset> <input type="submit" /> </form> <script type="text/javascript"> function myAction() { alert("Your information: \nName: " + $("#myName").val() + "\nTel: " + $("#telephone").val() + "\nemail: " + $("#email").val()); } </script>
This is produced by the following HTML5:
<!-- from css file: input:valid { background-color: #aaffaa; } input:invalid { background-color: #ffaaaa; } --> <form id="signUpForm2" autocomplete="on" action="javascript:howManyKittens();" method="get"> <fieldset> <legend> About Kittens </legend> <div> <label for="numberOfCats">How many cats do you already have?:</label> <input type="number" min="1" max="20" step="1" value="1" id="numberOfCats" name="numberOfCats" required> <br /><label for="numberOfKittens">How many kittens would you like each month? (1-6):</label> <input type="range" min="1" max="6" step="1" value="1" id="numberOfKittens" name="numberOfKittens"> <output class="kittens-output" for="numberOfKittens">101</output> <br /><label for="color">What color would you like?:</label><br /> <input type="color" id="color" name="color" /> </div> </fieldset> <input type="submit" /> <script> const numberOfKittensWidget = document.querySelector('#numberOfKittens'); const output = document.querySelector('.kittens-output'); output.textContent = numberOfKittensWidget.value; numberOfKittensWidget.addEventListener('input', function () { output.textContent = numberOfKittensWidget.value; }); </script> <script> function howManyKittens() { if(document.querySelector('.kittens-output').textContent > 4) { alert(numberOfKittensWidget.value + " is a lot of kittens!"); } else { alert("Come on, you need more than " + numberOfKittensWidget.value + "!"); } } </script> </form>
datalist is a new element which allows for a Google-suggest like experience.
This is produced by the following HTML5:
<form id="signUpForm3" autocomplete="on" action="javascript:myAction();" method="get"> <fieldset> <legend> More About Kittens </legend> <div> <br /><label for=typesOfCats>What is your favorite type of cat? (hint: tabby):</label> <input type="text" list=catList id=typesOfCats name=typesOfCats > <datalist id=catList> <option value=tabby> <option value=striped> <option value=solid> <option value=calico> <option value=polydactyl> </datalist> </div> </fieldset> </form>
If you want to set custom error messages you can use setCustomValidity().
Click here to join the Kitten-A-Month club today.